Introduction to REST and RESTful APIs
In the web development world, REST (Representational State Transfer) and RESTful APIs have become foundational concepts for building scalable, flexible, and maintainable web services. REST is an architectural style that defines a set of constraints for creating web services, while RESTful APIs are web services that adhere to the principles of REST. These APIs allow communication between client and server over HTTP, facilitating data exchange in a simple, standardized manner.
Benefits of RESTful APIs
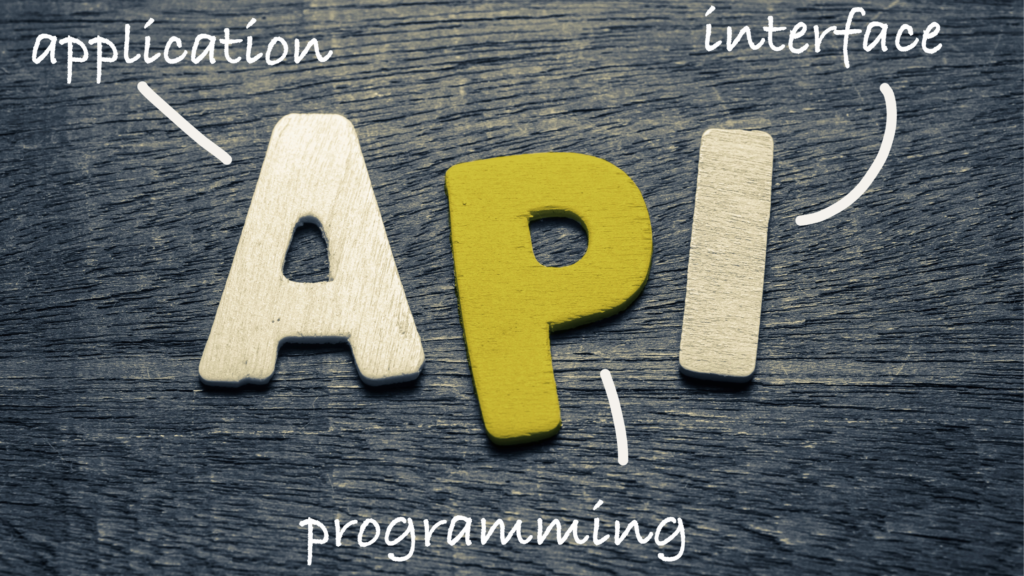
RESTful APIs have gained widespread popularity due to their numerous benefits:
- Scalability: RESTful APIs are stateless, meaning each request from a client to the server must contain all the information needed to understand and process the request. This makes scaling applications easier.
- Flexibility: RESTful APIs can handle different types of data formats, such as JSON, XML, and plain text, offering flexibility in communication.
- Performance: By using standard HTTP methods (GET, POST, PUT, DELETE), RESTful APIs can optimize the performance of applications by minimizing the amount of data transmitted between client and server.
- Interoperability: RESTful APIs allow seamless communication between different systems, regardless of the underlying technology stack.
Types of Web APIs
Web APIs can be categorized based on their architecture and design. The main types include:
- RESTful APIs: Follow the principles of REST and are widely used for web applications.
- SOAP APIs: Use the Simple Object Access Protocol to exchange structured information. They are more rigid and less flexible compared to RESTful APIs.
- GraphQL APIs: Provide a more flexible approach to querying data by allowing clients to specify exactly what data they need.
- RPC APIs: Use Remote Procedure Calls, either JSON-RPC or XML-RPC, to execute methods on the server.
REST API vs. API: Key Differences
While the term “API” (Application Programming Interface) refers to any set of protocols and tools for building software applications, a REST API is a specific type of API that adheres to the constraints of REST. Key differences include:
- Design Principles: REST APIs follow REST architectural principles, while other APIs may not.
- Communication: REST APIs use standard HTTP methods and are stateless, while other APIs may use different communication protocols.
- Data Formats: REST APIs typically use JSON or XML, while others might use SOAP, binary formats, or protocols.
Four Types of APIs
APIs can be broadly categorized into four types:
- Open APIs: Also known as external or public APIs, these are available to external developers and users with minimal restrictions.
- Internal APIs: Used within an organization, these APIs are not exposed to external users and are meant for internal use.
- Partner APIs: These APIs provide controlled access to services and data and are available to specific partners.
- Composite APIs: Allow the client to make a single API call to access multiple endpoints or services, simplifying complex client-server interactions.
RESTful API Design Methodology
Designing a RESTful API involves adhering to the following key principles:
- Resource Identification: Resources are identified by URIs (Uniform Resource Identifiers). For example, /users might represent a collection of users.
- Use of HTTP Methods: HTTP methods (GET, POST, PUT, DELETE) are used to perform actions on resources.
- GET retrieves data.
- POST creates new data.
- PUT updates existing data.
- DELETE removes data.
- Statelessness: Each request must contain all the information needed for the server to understand and process the request.
- Representation of Resources: Data is usually represented in JSON or XML format.
- HATEOAS: Hypermedia as the Engine of Application State (HATEOAS) allows the client to dynamically navigate the API based on links provided in the responses.
Sample Code Snippet: Basic RESTful API Design
<pre class="wp-block-code"><code>// Node.js Express example for a RESTful API const express = require('express'); const app = express(); app.use(express.json()); let users = [ { id: 1, name: 'John Doe' }, { id: 2, name: 'Jane Smith' } ]; // GET request to retrieve all users app.get('/users', (req, res) => { res.json(users); }); // POST request to add a new user app.post('/users', (req, res) => { const newUser = req.body; users.push(newUser); res.status(201).json(newUser); }); // PUT request to update a user app.put('/users/:id', (req, res) => { const userId = parseInt(req.params.id); const updatedUser = req.body; users = users.map(user => (user.id === userId ? updatedUser : user)); res.json(updatedUser); }); // DELETE request to delete a user app.delete('/users/:id', (req, res) => { const userId = parseInt(req.params.id); users = users.filter(user => user.id !== userId); res.status(204).send(); }); app.listen(3000, () => console.log('Server running on port 3000')); </code></pre>
Components of a RESTful API Client Request
A RESTful API client request typically includes the following components:
- Endpoint: The URL to which the request is made, identifying the resource.
- HTTP Method: Defines the type of operation (GET, POST, PUT, DELETE).
- Headers: Provide metadata for the request, such as Content-Type, Authorization, etc.
- Body: Contains data for POST and PUT requests, usually in JSON format.
RESTful API Authentication Methods
Securing RESTful APIs is crucial, and several authentication methods are commonly used:
- Basic Authentication: Involves sending the username and password encoded in the request header. It’s simple but less secure unless combined with HTTPS.
- Token-Based Authentication: After the client logs in with credentials, the server issues a token that the client uses for subsequent requests. Examples include JWT (JSON Web Tokens).
- OAuth: A more robust method that allows third-party services to exchange information on behalf of a user without sharing credentials. It’s widely used for integrating with external services.
- API Keys: Simple, often used for public APIs. An API key is passed in the request header or as a parameter to authenticate the request.
Pros and Cons of Authentication Methods
- Basic Authentication: Simple but vulnerable if not used with HTTPS.
- Token-Based Authentication: Secure and stateless, but token management can be complex.
- OAuth: Highly secure and flexible but more complex to implement.
- API Keys: Easy to use but less secure if not correctly managed.
Popular REST Clients
Several REST clients are popular among developers for testing and interacting with RESTful APIs:
- Postman: A powerful GUI-based tool for testing APIs with various features like environment variables, collections, and automated testing.
- Insomnia: A simple, user-friendly REST client with features like environment variables and support for GraphQL.
- cURL: A command-line tool for making HTTP requests that is useful for quick tests and automation.
- HTTPie: A command-line tool similar to cURL, with a more human-friendly syntax.
RESTful API vs. Open API
RESTful APIs and Open APIs (often referred to as public APIs) serve different purposes:
- RESTful API: A type of API architecture adhering to REST principles. It can be private, partner, or public.
- Open API: Refers to APIs that are publicly available, allowing external developers to access the API. They may or may not be RESTful.
Use Cases:
- RESTful API: Suitable for internal applications, partner integrations, or public use, depending on the design.
- Open API: Ideal for public-facing services, enabling third-party developers to build on top of your platform.
Conclusion
In this blog post, we have explored the fundamental concepts of REST and RESTful APIs, their benefits, and the different types of web APIs. We delved into the design principles of RESTful APIs, examined various authentication methods, and highlighted popular tools for interacting with these APIs.
Understanding REST and RESTful APIs is crucial for modern web development, as they form the backbone of communication between web services and applications. Whether building a simple application or integrating complex systems, RESTful APIs offer a standardized approach to creating scalable and maintainable web services. You should explore and implement RESTful APIs in your projects to enhance your development skills and build more efficient applications. Here is a good article to learn more about API reusability
Leave A Reply